You can manage large datasets and dynamic content with lazy grids, improving app performance. With lazy grid composables, you can display items in a scrollable container, spanned across multiple columns or rows.
Version compatibility
This implementation requires that your project minSDK be set to API level 21 or higher.
Dependencies
Decide grid orientation
The LazyHorizontalGrid
and LazyVerticalGrid
composables provide
support for displaying items in a grid. A lazy vertical grid displays its items
in a vertically scrollable container, spanned across multiple columns, while
lazy horizontal grids have the same behavior on the horizontal axis.
Create a scrollable grid
The following code creates a horizontal scrolling grid with three columns:
Key points about the code
- The
LazyHorizontalGrid
composable determines the horizontal orientation of the grid.- To create a vertical grid, use the
LazyVerticalGrid
instead.
- To create a vertical grid, use the
- The
rows
property specifies how to arrange the grid content.- For a vertical grid, use the
columns
property to specify the arrangement.
- For a vertical grid, use the
items(itemsList)
addsitemsList
toLazyHorizontalGrid
. The lambda renders aText
composable for each item and set the text to the item description.item()
adds a single item toLazyHorizontalGrid
while the lambda renders a singleText
composable in a similar manner toitems()
.GridCells.Fixed
defines the number of rows or columns.To create a grid with as many rows as possible, set the number of rows using
GridCells.Adaptive
.In the following code, the
20.dp
value specifies that every column is at least 20.dp, and all columns have equal widths. If the screen is 88.dp wide, there are 4 columns at 22.dp each.
Results
LazyHorizontalGrid
.Collections that contain this guide
This guide is part of these curated Quick Guide collections that cover broader Android development goals:
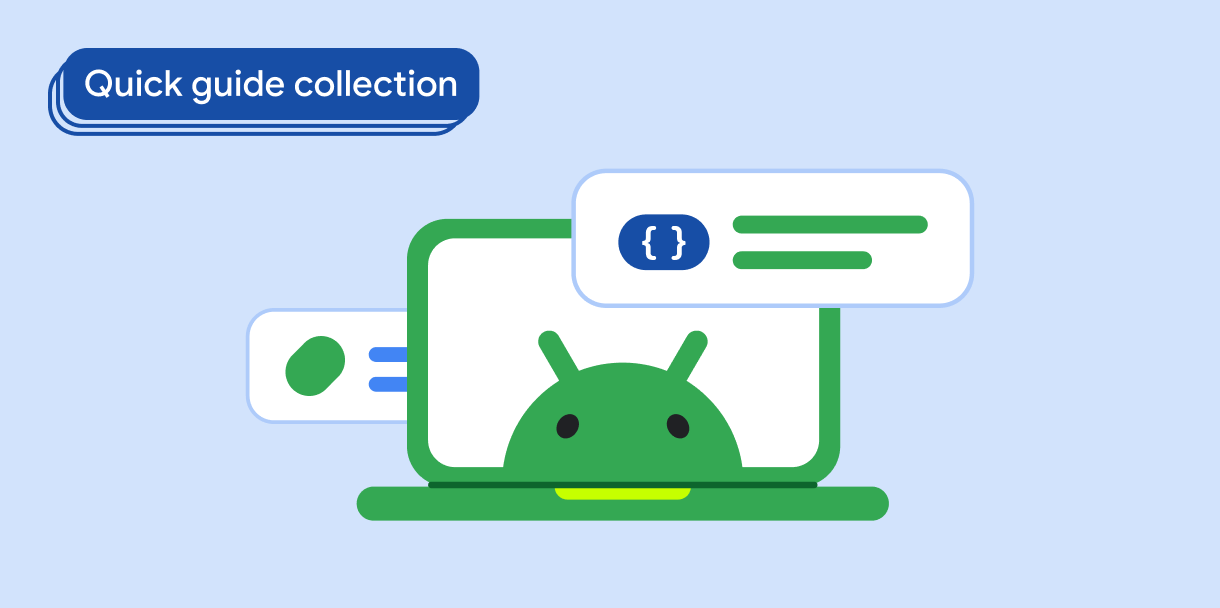
Display a list or grid
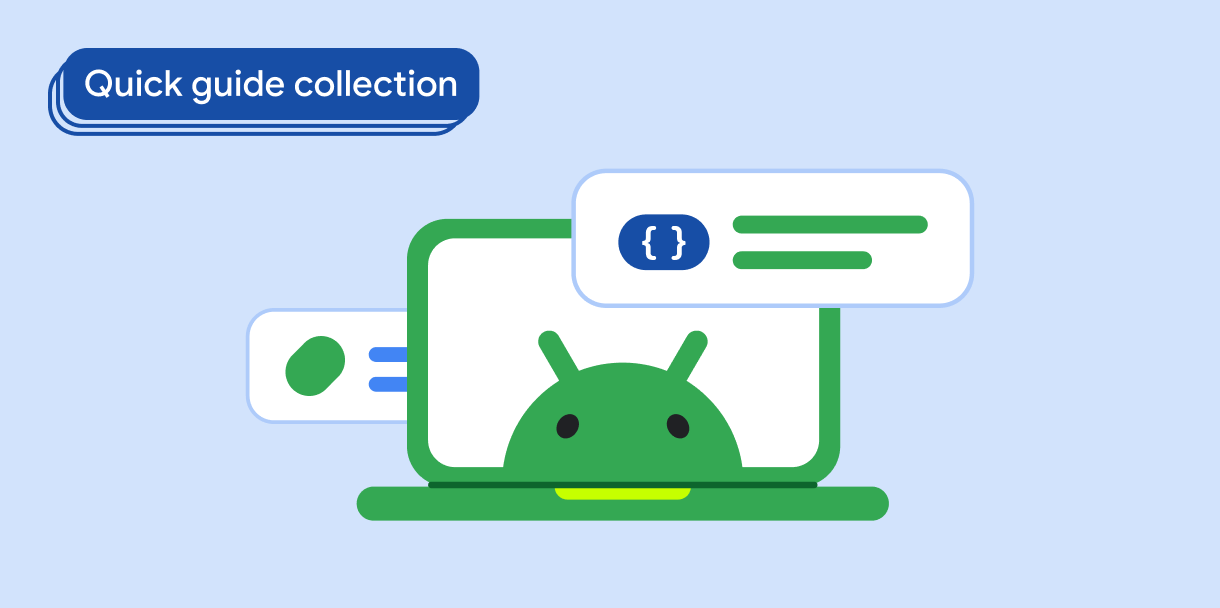
Display interactive components
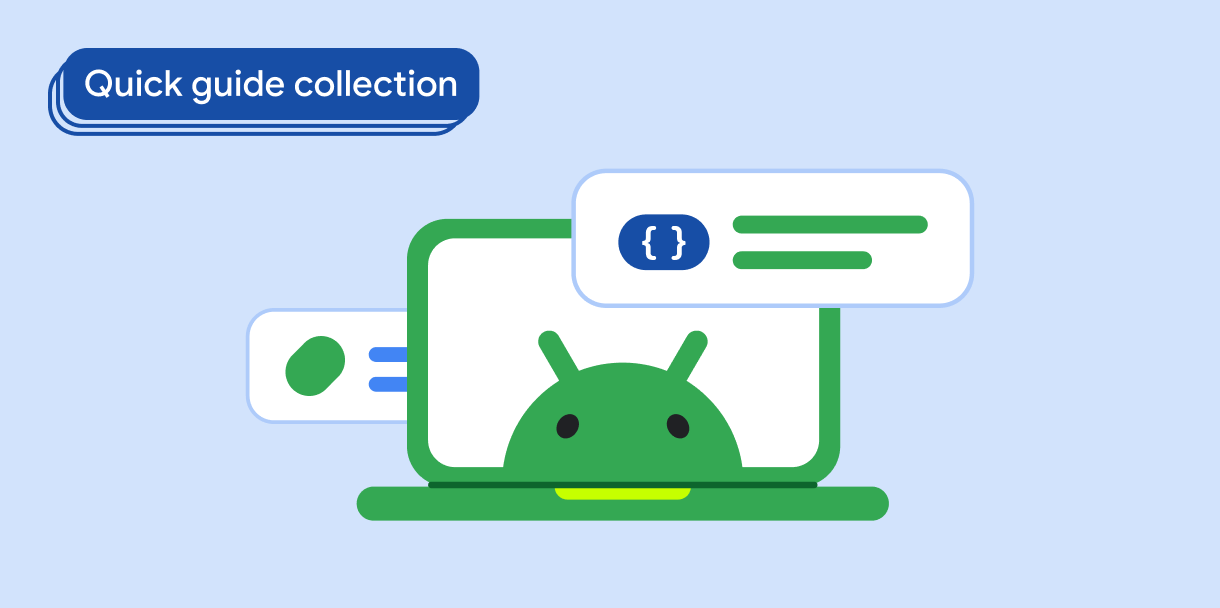