WebView
is a commonly used
component that offers an advanced system for state management. A WebView
must maintain its state and scroll position across configuration changes. A
WebView
can lose scroll position when the user rotates the device or unfolds
a foldable phone, which forces the user to scroll again from the top of the
WebView
to the previous scroll position.
WebView
is good at managing its state. You can take advantage of this quality
by managing as many configuration changes as possible to minimize the number of
times a WebView
is recreated. Your app should handle configuration changes
because activity recreation (the system's way of handling configuration
changes) recreates the WebView
, which causes the WebView
to lose state.
Manage state
Avoid Activity
recreation as much as
possible during configuration changes, and let the WebView
invalidate so it
can resize while retaining its state.
To manage WebView
state:
- Declare configuration changes handled by your app
- Invalidate the
WebView
state
1. Add configuration changes to your app's AndroidManifest.xml
file
Avoid activity recreation by specifying the configuration changes handled by your app (rather than by the system):
<activity
android:name=".MyActivity"
android:configChanges="screenLayout|orientation|screenSize
|keyboard|keyboardHidden|smallestScreenSize" />
2. Invalidate WebView
whenever your app receives a configuration change
Kotlin
override fun onConfigurationChanged(newConfig: Configuration) { super.onConfigurationChanged(newConfig) webView.invalidate() }
Java
@Override public void onConfigurationChanged(@NonNull Configuration newConfig) { super.onConfigurationChanged(newConfig); webview.invalidate(); }
This step applies only to the view system, as Jetpack Compose does not need to
invalidate anything to resize
Composable
elements
correctly. However, Compose recreates a WebView
often if not managed
correctly. Use the
Accompanist WebView
wrapper to save and restore WebView
state in your Compose apps.
Key points
android:configChanges
: Attribute of the manifest<activity>
element. Lists the configuration changes handled by the activity.View#invalidate()
: Method that causes a view to be redrawn. Inherited byWebView
.
Results
Your app's WebView
components now retain their state and scroll position
across multiple configuration changes, from resizing to orientation changes
to device folding and unfolding.
Collections that contain this guide
This guide is part of these curated Quick Guide collections that cover broader Android development goals:
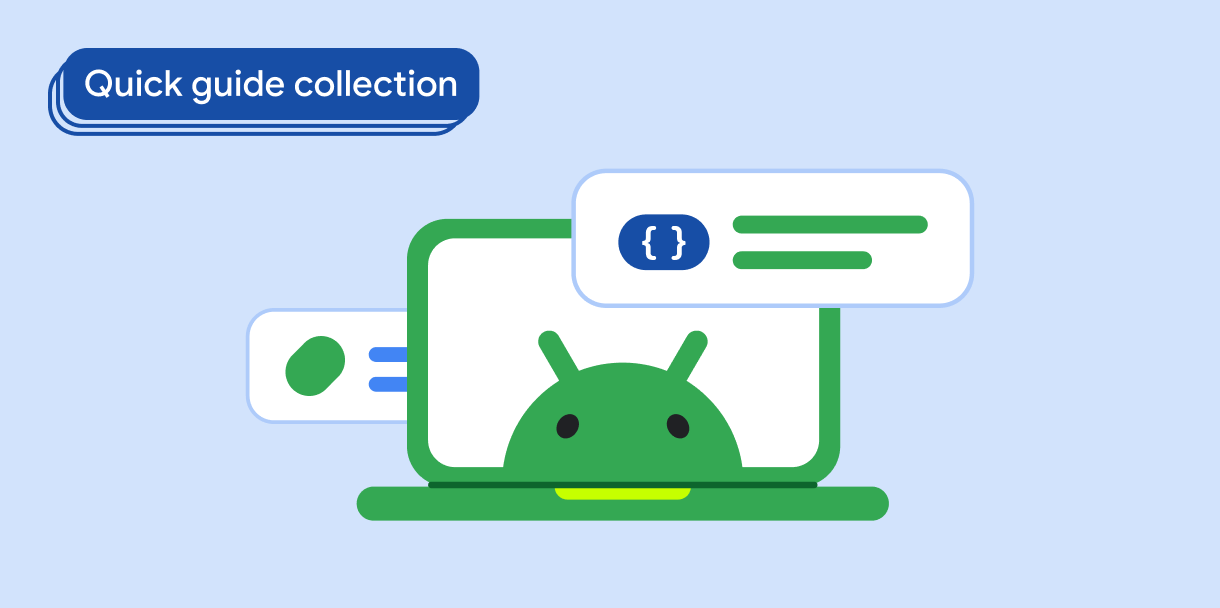