您的应用可能具有多步骤用户任务。例如,您的应用可能需要引导用户购买其他内容、设置复杂的配置设置,或者只是确认决定。所有这些任务都需要引导用户完成一个或多个有序的步骤或决策。
Leanback androidx 库提供了用于实现多步骤用户任务的类。本页介绍了如何使用 GuidedStepSupportFragment
类引导用户完成一系列决定,以便完成任务。GuidedStepSupportFragment
利用 TV 界面最佳实践,让多步骤任务在 TV 设备上易于理解和导航。
提供步骤详情
GuidedStepSupportFragment
表示一系列步骤中的单个步骤。它直观地提供了一个指导视图,其中包含该步骤可能执行的操作或决定的列表。
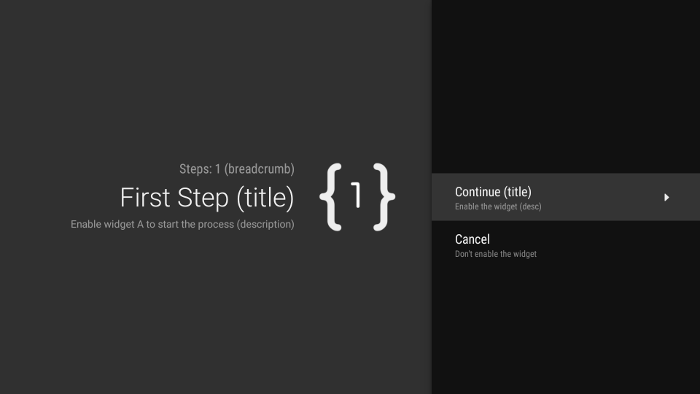
图 1. 引导步骤示例。
对于多步骤任务中的每个步骤,请扩展 GuidedStepSupportFragment
并提供有关用户可以执行的步骤和操作的上下文信息。替换 onCreateGuidance()
并返回包含上下文信息(例如步骤标题、说明和图标)的新 GuidanceStylist.Guidance
,如以下示例所示:
Kotlin
override fun onCreateGuidance(savedInstanceState: Bundle?): GuidanceStylist.Guidance { return GuidanceStylist.Guidance( getString(R.string.guidedstep_first_title), getString(R.string.guidedstep_first_description), getString(R.string.guidedstep_first_breadcrumb), activity.getDrawable(R.drawable.guidedstep_main_icon_1) ) }
Java
@Override public GuidanceStylist.Guidance onCreateGuidance(Bundle savedInstanceState) { String title = getString(R.string.guidedstep_first_title); String breadcrumb = getString(R.string.guidedstep_first_breadcrumb); String description = getString(R.string.guidedstep_first_description); Drawable icon = getActivity().getDrawable(R.drawable.guidedstep_main_icon_1); return new GuidanceStylist.Guidance(title, description, breadcrumb, icon); }
通过在 activity 的 onCreate()
方法中调用 GuidedStepSupportFragment.add()
,您可以将 GuidedStepSupportFragment
子类添加到所需的 activity。
如果 activity 仅包含 GuidedStepSupportFragment
对象,请使用 GuidedStepSupportFragment.addAsRoot()
(而非 add()
)添加第一个 GuidedStepSupportFragment
。使用 addAsRoot()
有助于确保用户在查看第一个 GuidedStepSupportFragment
时按下电视遥控器上的返回按钮,GuidedStepSupportFragment
和父 activity 都会关闭。
注意:以编程方式添加 GuidedStepSupportFragment
对象,而不是在布局 XML 文件中添加。
创建和处理用户操作
通过替换 onCreateActions()
添加用户操作。在替换项中,为每个操作项添加新的 GuidedAction
,并提供操作字符串、说明和 ID。使用 GuidedAction.Builder
添加新操作。
Kotlin
override fun onCreateActions(actions: MutableList<GuidedAction>, savedInstanceState: Bundle?) { super.onCreateActions(actions, savedInstanceState) // Add "Continue" user action for this step actions.add(GuidedAction.Builder() .id(CONTINUE) .title(getString(R.string.guidedstep_continue)) .description(getString(R.string.guidedstep_letsdoit)) .hasNext(true) .build()) ...
Java
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { // Add "Continue" user action for this step actions.add(new GuidedAction.Builder() .id(CONTINUE) .title(getString(R.string.guidedstep_continue)) .description(getString(R.string.guidedstep_letsdoit)) .hasNext(true) .build()); ...
操作不仅限于单行选择。以下是您可以创建的其他类型的操作:
-
通过设置
infoOnly(true)
添加信息标签操作,以提供有关用户选择的更多信息。如果infoOnly
为 true,则用户无法选择操作。 -
通过设置
editable(true)
添加可编辑的文本操作。当editable
为 true 时,用户可以使用遥控器或已连接的键盘在所选操作中输入文本。替换onGuidedActionEditedAndProceed()
以获取用户输入的修改后的文本。您还可以替换onGuidedActionEditCanceled()
以了解用户何时取消输入。 -
使用具有通用 ID 值的
checkSetId()
将操作分组到一个组中,从而添加一组行为类似于可选中单选按钮的操作。同一列表中具有相同勾选组 ID 的所有操作均被视为已关联。当用户选择该组内的某项操作时,该操作将变为未选中状态,而所有其他操作则变为未选中状态。 -
在
onCreateActions()
中使用GuidedDatePickerAction.Builder
(而不是GuidedAction.Builder
)添加日期选择器操作。替换onGuidedActionEditedAndProceed()
以获取用户输入的修改后的日期值。 - 添加使用子操作的操作,以便用户从扩展选择列表中进行选择。添加子操作部分介绍了子操作。
- 添加显示在操作列表右侧且可轻松访问的按钮操作。添加按钮操作部分介绍了按钮操作。
您还可以通过设置 hasNext(true)
添加一个可见指示符,指示选择某项操作后会转到新步骤。
如需了解您可以设置的所有不同属性,请参阅 GuidedAction
。
如需响应操作,请替换 onGuidedActionClicked()
并处理传入的 GuidedAction
。通过检查 GuidedAction.getId()
确定所选操作。
添加子操作
某些操作可能需要您为用户提供一组额外的选项。GuidedAction
可以指定以子操作菜单的形式显示的子操作列表。
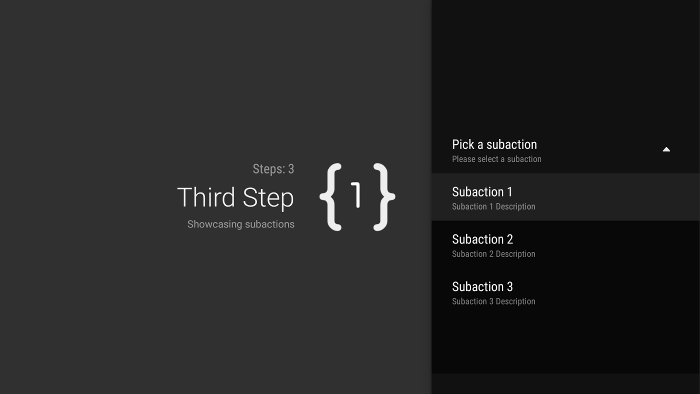
图 2. 引导步骤子操作。
子操作列表可以包含常规操作或单选按钮操作,但不包含日期选择器或可修改的文本操作。此外,子操作也不能拥有自己的一组子操作,因为系统不支持超过一个级别的子操作。
如需添加子操作,请先创建并填充充当子操作的 GuidedAction
对象列表,如以下示例所示:
Kotlin
subActions.add(GuidedAction.Builder() .id(SUBACTION1) .title(getString(R.string.guidedstep_subaction1_title)) .description(getString(R.string.guidedstep_subaction1_desc)) .build()) ...
Java
List<GuidedAction> subActions = new ArrayList<GuidedAction>(); subActions.add(new GuidedAction.Builder() .id(SUBACTION1) .title(getString(R.string.guidedstep_subaction1_title)) .description(getString(R.string.guidedstep_subaction1_desc)) .build()); ...
在 onCreateActions()
中,创建一个顶级 GuidedAction
,以显示选中的子操作列表:
Kotlin
... actions.add(GuidedAction.Builder() .id(SUBACTIONS) .title(getString(R.string.guidedstep_subactions_title)) .description(getString(R.string.guidedstep_subactions_desc)) .subActions(subActions) .build()) ...
Java
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { ... actions.add(new GuidedAction.Builder() .id(SUBACTIONS) .title(getString(R.string.guidedstep_subactions_title)) .description(getString(R.string.guidedstep_subactions_desc)) .subActions(subActions) .build()); ... }
最后,通过替换 onSubGuidedActionClicked()
来响应子操作选择:
Kotlin
override fun onSubGuidedActionClicked(action: GuidedAction): Boolean { // Check for which action was clicked and handle as needed when(action.id) { SUBACTION1 -> { // Subaction 1 selected } } // Return true to collapse the subactions menu or // false to keep the menu expanded return true }
Java
@Override public boolean onSubGuidedActionClicked(GuidedAction action) { // Check for which action was clicked and handle as needed if (action.getId() == SUBACTION1) { // Subaction 1 selected } // Return true to collapse the subactions menu or // false to keep the menu expanded return true; }
添加按钮操作
如果您的引导步骤包含大量操作,用户可能必须滚动浏览该列表才能访问最常用的操作。使用按钮操作将常用操作与操作列表分开。按钮操作显示在操作列表旁边,并且易于导航。
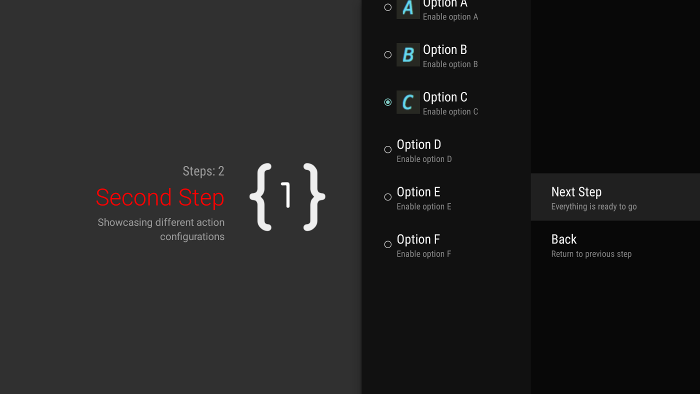
图 3. 引导步骤按钮操作。
按钮操作的创建和处理方式与常规操作一样,但您可以在 onCreateButtonActions()
(而不是 onCreateActions()
)中创建按钮操作。响应 onGuidedActionClicked()
中的按钮操作。
将按钮操作用于简单操作,例如步骤之间的导航操作。 请勿将日期选择器操作或其他可修改操作用作按钮操作。此外,按钮操作不能包含子操作。
将引导步骤分组到引导序列中
GuidedStepSupportFragment
表示一步。如需创建有序的步骤序列,请使用 GuidedStepSupportFragment.add()
将多个 GuidedStepSupportFragment
对象分组在一起,以将序列中的下一步添加到 fragment 堆栈。
Kotlin
override fun onGuidedActionClicked(action: GuidedAction) { val fm = fragmentManager when(action.id) { CONTINUE -> GuidedStepSupportFragment.add(fm, SecondStepFragment()) } }
Java
@Override public void onGuidedActionClicked(GuidedAction action) { FragmentManager fm = getFragmentManager(); if (action.getId() == CONTINUE) { GuidedStepSupportFragment.add(fm, new SecondStepFragment()); } ...
如果用户按电视遥控器上的返回按钮,设备会在 fragment 堆栈上显示上一个 GuidedStepSupportFragment
。如果您提供自己的 GuidedAction
(用于返回到上一步),则可以通过调用 getFragmentManager().popBackStack()
来实现“返回”行为。
如果您需要让用户返回到序列中更早的步骤,请使用 popBackStackToGuidedStepSupportFragment()
返回到 fragment 堆栈中的特定 GuidedStepSupportFragment
。
当用户完成序列中的最后一步时,使用 finishGuidedStepSupportFragments()
从当前堆栈中移除所有 GuidedStepSupportFragment
实例并返回到原始父 activity。如果使用 addAsRoot()
添加第一个 GuidedStepSupportFragment
,则调用 finishGuidedStepSupportFragments()
也会关闭父 activity。
自定义步骤呈现
GuidedStepSupportFragment
类可以使用自定义主题来控制呈现方面的内容,例如标题文本格式设置或分步过渡动画。自定义主题必须继承自 Theme_Leanback_GuidedStep
,并且可以为 GuidanceStylist
和 GuidedActionsStylist
中定义的属性提供替换值。
如需将自定义主题应用于 GuidedStepSupportFragment
,请执行以下操作之一:
-
通过将
android:theme
属性设置为 Android 清单中的 activity 元素,将主题应用于父 activity。设置此属性会将主题应用于所有子视图,如果父 activity 仅包含GuidedStepSupportFragment
对象,则是应用自定义主题的最直接方法。 -
如果您的 activity 已在使用自定义主题,并且您不希望将
GuidedStepSupportFragment
样式应用于 activity 中的其他视图,请将LeanbackGuidedStepTheme_guidedStepTheme
属性添加到现有的自定义 activity 主题中。此属性指向只有 activity 中的GuidedStepSupportFragment
对象使用的自定义主题。 -
如果您在属于同一整体多步骤任务的不同 activity 中使用
GuidedStepSupportFragment
对象,并且希望在所有步骤中使用一致的视觉主题,请替换GuidedStepSupportFragment.onProvideTheme()
并返回您的自定义主题。
如需详细了解如何添加样式和主题,请参阅样式和主题。
GuidedStepSupportFragment
类使用特殊的样式器类来访问和应用主题属性。GuidanceStylist
类使用主题信息来控制左侧导航视图的呈现,而 GuidedActionsStylist
类使用主题信息控制右侧操作视图的呈现。
如需自定义主题自定义所提供步骤之外的视觉样式,请创建 GuidanceStylist
或 GuidedActionsStylist
的子类,并返回 GuidedStepSupportFragment.onCreateGuidanceStylist()
或 GuidedStepSupportFragment.onCreateActionsStylist()
中的子类。如需详细了解您可以在这些子类中自定义的内容,请参阅有关 GuidanceStylist
和 GuidedActionsStylist
的文档。