构建于 Device Discovery 和 Secure Connection API 之上, Sessions API 提供了一个强大的抽象功能,用于构建无缝的跨设备 体验。会话表示应用的用户体验, 在不同设备之间传输和共享的数据。
Sessions API 也是围绕个人和公共的概念构建的。 会话转移和会话共享用例所代表的体验 。下图概括展示了会话:
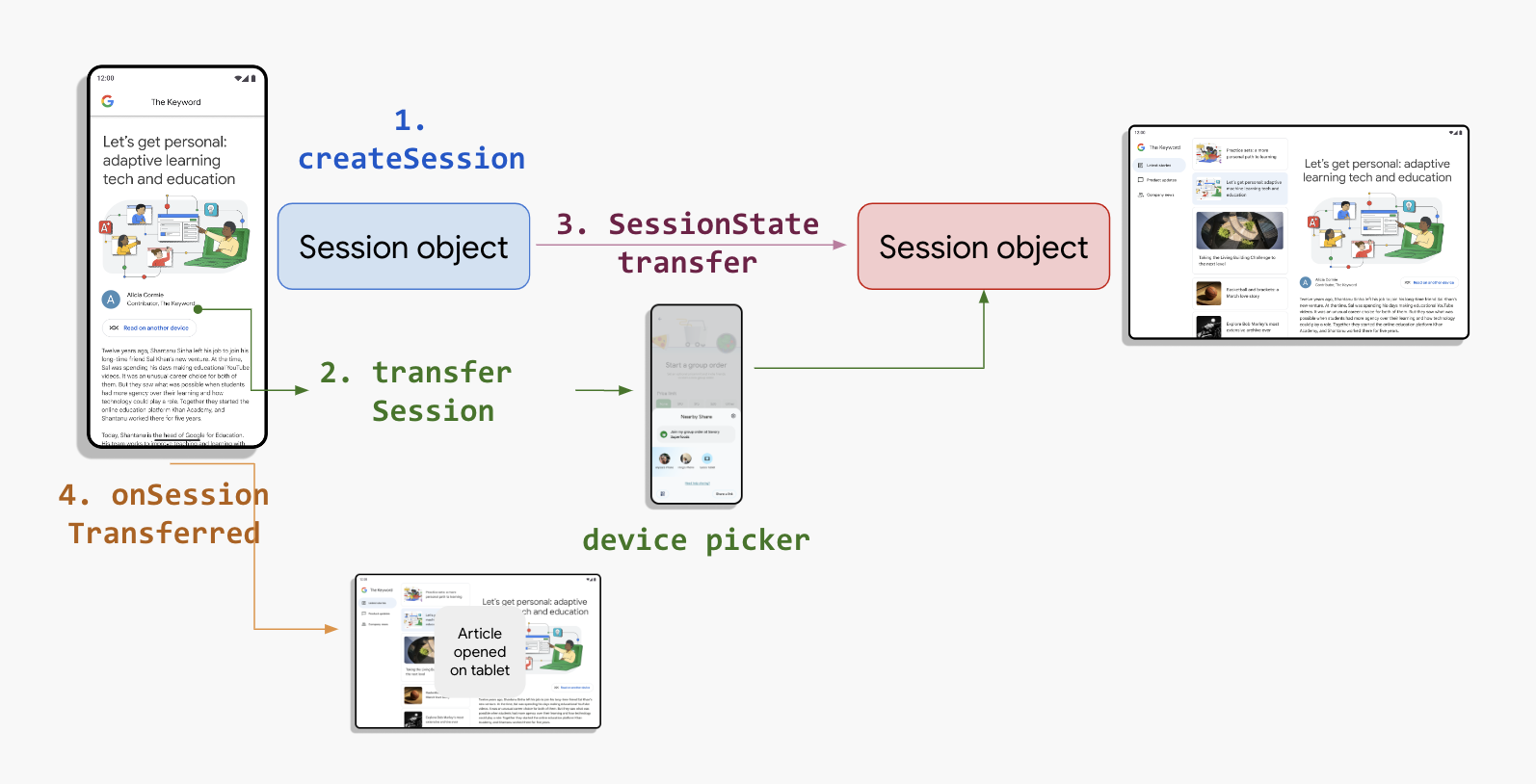
创建和转移会话
Sessions API 用于区分源设备和接收设备。源设备会创建会话并搜索 能够处理会话的设备。源设备的用户 用于从系统对话框提供的列表中选择设备。用户 选择接收设备,源会话会被转移并移除 发出电子邮件请求。
如需转移会话,您必须先使用以下命令创建会话 参数:
- 应用会话标记 - 可让您区分 在不同会话间切换
然后,使用以下参数启动传输:
DeviceFilter
,用于过滤能够处理会话的设备- 实现
OriginatingSessionStateCallback
的回调对象
在源设备上,按照以下示例创建会话:
Kotlin
private val HELLO_WORLD_TRANSFER_ACTION = "hello_world_transfer" private lateinit var originatingSession: OriginatingSession private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } suspend fun transferSession() { val sessionId = sessions.createSession( ApplicationSessionTag("hello_world_transfer"), ) originatingSession = sessions.transferSession( sessionId, StartComponentRequest.Builder() .setAction(HELLO_WORLD_TRANSFER_ACTION) .setReason("Transfer reason here") .build(), emptyList(), HelloWorldTransferSessionStateCallback() ) }
Java
private static final String HELLO_WORLD_TRANSFER_ACTION = "hello_world_transfer"; private OriginatingSession originatingSession; private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } public void transferSession() { SessionId sessionId = sessions.createSession(new ApplicationSessionTag("hello_world_transfer")); ListenableFuture<OriginatingSession> originatingSessionFuture = sessions.transferSessionFuture( sessionId, new StartComponentRequest.Builder() .setAction(HELLO_WORLD_TRANSFER_ACTION) .setReason("Transfer reason here") .build(), Collections.emptyList(), new HelloWorldTransferSessionStateCallback()); Futures.addCallback( originatingSessionFuture, new FutureCallback<>() { @Override public void onSuccess(OriginatingSession result) { // Do nothing, handled in HelloWorldTransferSessionStateCallback originatingSession = result; } @Override public void onFailure(Throwable t) { Log.d(TAG, "onFailure called for transferSessionFuture", t); } }, mainExecutor); }
接下来,在源设备上定义会话回调:
Kotlin
private inner class HelloWorldTransferSessionStateCallback : OriginatingSessionStateCallback { override fun onConnected(sessionId: SessionId) { val startupRemoteConnection = originatingSession.getStartupRemoteConnection() lifecycleScope.launchWhenResumed { startupRemoteConnection.send("hello, world".toByteArray(UTF_8)) startupRemoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { val ok = payload.contentEquals("ok".toByteArray(UTF_8)) Log.d(TAG, "Session transfer initialized. ok=$ok") } } ) } } override fun onSessionTransferred(sessionId: SessionId) { Log.d(TAG, "Transfer done.") } override fun onTransferFailure(sessionId: SessionId, exception: SessionException) { // Handle error } }
Java
private class HelloWorldTransferSessionStateCallback implements OriginatingSessionStateCallback { @Override public void onConnected(SessionId sessionId) { SessionRemoteConnection startupRemoteConnection = originatingSession.getStartupRemoteConnection(); Futures.addCallback( startupRemoteConnection.sendFuture("hello, world".getBytes()), new FutureCallback<>() { @Override public void onSuccess(Void result) { Log.d(TAG, "Successfully sent initialization message"); } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to send initialization message", t); } }, mainExecutor); } @Override public void onSessionTransferred(SessionId sessionId) { Log.d(TAG, "Transfer done."); } @Override public void onTransferFailure(SessionId sessionId, SessionException exception) { // Handle error } }
启动会话传输后,接收方设备将收到回调
(位于 onNewIntent(intent: Intent)
方法中)。intent 数据包含所有
传输会话所需的资源。
如需在接收设备上完成转移,请执行以下操作:
Kotlin
private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) lifecycleScope.launchWhenResumed { val receivingSession = sessions.getReceivingSession(intent, HelloWorldReceivingSessionStateCallback()) // Get info from receiving device and init. val startupRemoteConnection = receivingSession.getStartupRemoteConnection() startupRemoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { lifecycleScope.launchWhenResumed { val transferInfo = String(payload) startupRemoteConnection.send("ok".toByteArray(UTF_8)) // Complete transfer. Log.d(TAG, "Transfer info: " + transferInfo) receivingSession.onComplete() } } } ) } } private inner class HelloWorldReceivingSessionStateCallback : ReceivingSessionStateCallback { override fun onTransferFailure(sessionId: SessionId, exception: SessionException) { // Handle error } }
Java
private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); ListenableFuture<ReceivingSession> receivingSessionFuture = sessions.getReceivingSessionFuture(intent, new HelloWorldReceivingSessionStateCallback()); ListenableFuture<Void> registerReceiverFuture = Futures.transform( receivingSessionFuture, receivingSession -> { SessionRemoteConnection startupRemoteConnection = receivingSession.getStartupRemoteConnection(); SessionConnectionReceiver receiver = (participant, payload) -> { Log.d( TAG, "Successfully received initialization message of size: " + payload.length); applicationInitialization(receivingSession, payload); }; startupRemoteConnection.registerReceiver(receiver); return null; }, mainExecutor); Futures.addCallback( registerReceiverFuture, new FutureCallback<Void>() { @Override public void onSuccess(Void unused) { Log.d(TAG, "Connection receiver registerd successfully"); } @Override public void onFailure(Throwable t) { Log.w(TAG, "Failed to register connection receiver", t); } }, mainExecutor); } private void applicationInitialization(ReceivingSession receivingSession, byte[] initMessage) { ListenableFuture<SessionId> disconnectFuture = Futures.transform( receivingSession.onCompleteFuture(), sessionId -> { Log.d(TAG, "Succeeded to complete receive transfer for: " + sessionId); return sessionId; }, mainExecutor); Futures.addCallback( disconnectFuture, new FutureCallback<SessionId>() { @Override public void onSuccess(SessionId result) { Log.d(TAG, "Succeeded to remove the old session: " + result); } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to remove the old session, which is now orphaned", t); } }, mainExecutor); } private static class HelloWorldReceivingSessionStateCallback implements ReceivingSessionStateCallback { @Override public void onTransferFailure(SessionId sessionId, SessionException exception) { // Handle error } }
现在,接收设备可以继续提供用户体验了。
分享会话
通过分享会话,您可以邀请周围的其他人加入群组 例如:
- 作为乘客,直接与好友的汽车分享地图位置。
- 与其他骑行者分享您的周日骑车路线。
- 无需四处走动,即可领取团体订购的食物。
- 进行小组投票,选出要一起观看的下一部电视剧。
当用户选择与其他设备共享会话时,源设备 设备会搜索并显示能够加入会话的设备, 用户选择接收设备。应用提示用户 接收方设备加入会话。接收方 然后为设备提供次要会话,以与设备上的会话进行互动 发起请求。应用还可以将其他参与者添加到 进行中的共享会话。
共享会话的流程与转移会话类似,
但调用 shareSession
,而不是调用 transferSession
。另一个
主要区别在于会话状态回调方法。
Kotlin
// Originating side. private val HELLO_WORLD_SHARE_ACTION = "hello_world_share" private var activePrimarySession: PrimarySession? = null private lateinit var sessions: Sessions override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) sessions = Sessions.create(context = this) } suspend fun shareSession() { val sessionId = sessions.createSession(ApplicationSessionTag("hello_world_share")) activePrimarySession = sessions.shareSession( sessionId, StartComponentRequest.Builder() .setAction(HELLO_WORLD_SHARE_ACTION) .setReason("Share reason here") .build(), emptyList(), HelloWorldShareSessionStateCallback(), ) } private inner class HelloWorldShareSessionStateCallback : PrimarySessionStateCallback { override fun onShareInitiated(sessionId: SessionId, numPotentialParticipants: Int) { // Custom logic here for when n devices can potentially join. // e.g. if there were 0, cancel/error if desired, // if non-0 maybe spin until numPotentialParticipants join etc. } override fun onParticipantJoined(sessionId: SessionId, participant: SessionParticipant) { // Custom join logic here lifecycleScope.launchWhenResumed { // Example logic: send only to the participant who just joined. val connection = checkNotNull(activePrimarySession).getSecondaryRemoteConnectionForParticipant(participant) connection.send("Initing hello, world.".toByteArray(UTF_8)) connection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { val ok = payload.contentEquals("ok".toByteArray(UTF_8)) Log.d(TAG, "Session share initialized. ok=$ok") // Example logic: broadcast to all participants, including the one // that just joined. lifecycleScope.launchWhenResumed { checkNotNull(activePrimarySession) .broadcastToSecondaries("hello, all.".toByteArray(UTF_8)) } } } ) } } override fun onParticipantDeparted(sessionId: SessionId, participant: SessionParticipant) { // Custom leave logic here. } override fun onPrimarySessionCleanup(sessionId: SessionId) { // Custom cleanup logic here. activePrimarySession = null } override fun onShareFailureWithParticipant( sessionId: SessionId, exception: SessionException, participant: SessionParticipant ) { // Handle error } }
Java
// Originating side private static final String HELLO_WORLD_SHARE_ACTION = "hello_world_share"; @Nullable private PrimarySession activePrimarySession = null; private Sessions sessions; @Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); sessions = Sessions.create(/* context= */ this); } private void shareSession() { SessionId sessionId = sessions.createSession(new ApplicationSessionTag("hello_world_share")); ListenableFuture<PrimarySession> shareSessionFuture = sessions.shareSessionFuture( sessionId, new StartComponentRequest.Builder() .setAction(HELLO_WORLD_SHARE_ACTION) .setReason("Share reason here") .build(), Collections.emptyList(), new HelloWorldShareSessionStateCallback()); Futures.addCallback( shareSessionFuture, new FutureCallback<PrimarySession>() { @Override public void onSuccess(PrimarySession primarySession) { activePrimarySession = primarySession; } @Override public void onFailure(Throwable t) { Log.d(TAG, "Failed to share session", t); } }, mainExecutor); } private class HelloWorldShareSessionStateCallback implements PrimarySessionStateCallback { @Override public void onShareInitiated(SessionId sessionId, int numPotentialParticipants) { // Custom logic here for when n devices can potentially join. // e.g. if there were 0, cancel/error if desired, // if non-0 maybe spin until numPotentialParticipants join etc. } @Override public void onParticipantJoined(SessionId sessionId, SessionParticipant participant) { PrimarySession joinedSession = activePrimarySession; if (joinedSession == null) { return; } SessionRemoteConnection connection = joinedSession.getSecondaryRemoteConnectionForParticipant(participant); Futures.addCallback( connection.sendFuture("Initiating hello, world.".getBytes()), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // Send successful. } @Override public void onFailure(Throwable t) { // Failed to send. } }, mainExecutor); connection.registerReceiver( new SessionConnectionReceiver() { @Override public void onMessageReceived(SessionParticipant participant, byte[] payload) { boolean ok = new String(payload, UTF_8).equals("ok"); Log.d(TAG, "Session share initialized. ok=" + ok); // Example logic: broadcast to all participants, including the one // that just joined. Futures.addCallback( joinedSession.broadcastToSecondariesFuture("hello, all.".getBytes()), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // Broadcast successful. } @Override public void onFailure(Throwable t) { // Failed to broadcast hello world. } }, mainExecutor); } }); } @Override public void onParticipantDeparted(SessionId sessionId, SessionParticipant participant) { // Custom leave logic here. } @Override public void onPrimarySessionCleanup(SessionId sessionId) { // Custom cleanup logic here. activePrimarySession = null; } @Override public void onShareFailureWithParticipant( SessionId sessionId, SessionException exception, SessionParticipant participant) { // Custom error handling logic here. } }
在接收侧执行的操作:
Kotlin
// Receiving side. override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) lifecycleScope.launchWhenResumed { val secondarySession = sessions.getSecondarySession(intent, HelloWorldSecondaryShareSessionStateCallback()) val remoteConnection = secondarySession.getDefaultRemoteConnection() remoteConnection.registerReceiver( object : SessionConnectionReceiver { override fun onMessageReceived(participant: SessionParticipant, payload: ByteArray) { Log.d(TAG, "Payload received: ${String(payload)}") } } ) } } private inner class HelloWorldSecondaryShareSessionStateCallback : SecondarySessionStateCallback { override fun onSecondarySessionCleanup(sessionId: SessionId) { // Custom cleanup logic here. } }
Java
// Receiving side. @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); sessions = Sessions.create(this); ListenableFuture<SecondarySession> secondarySessionFuture = sessions.getSecondarySessionFuture( intent, new HelloWorldSecondaryShareSessionStateCallback()); Futures.addCallback( secondarySessionFuture, new FutureCallback<SecondarySession>() { @Override public void onSuccess(SecondarySession secondarySession) { SessionRemoteConnection remoteConnection = secondarySession.getDefaultRemoteConnection(); remoteConnection.registerReceiver( new SessionConnectionReceiver() { @Override public void onMessageReceived(SessionParticipant participant, byte[] payload) { Log.d(TAG, "Payload received: " + new String(payload, UTF_8)); } }); } @Override public void onFailure(Throwable t) { // Handle error. } }, mainExecutor); } private static class HelloWorldSecondaryShareSessionStateCallback implements SecondarySessionStateCallback { @Override public void onSecondarySessionCleanup(SessionId sessionId) { // Custom cleanup logic here. } }