为了让您的通知在不同的 Android 版本上都能达到最佳显示效果, 请使用标准通知 模板来构建自己的 通知。如果您想在通知中提供更多内容, 那么您可以考虑使用一种展开式通知 模板。
但是,如果系统模板不能满足您的需求,您可以使用自己的 通知的布局
为内容区域创建自定义布局
如果您需要自定义布局,
NotificationCompat.DecoratedCustomViewStyle
。您可以使用此 API 为内容提供自定义布局。
通常由标题和文本内容占据,同时仍然使用
通知图标、时间戳、辅助文本和操作按钮的装饰。
此 API 的运作方式与展开式通知模板类似,都是以基本通知为基础构建而成。 布局如下所示:
- 构建基本通知
替换为
NotificationCompat.Builder
。 - 致电
setStyle()
、 并向其传递NotificationCompat.DecoratedCustomViewStyle
。 - 将自定义布局扩充为
RemoteViews
。 - 致电
setCustomContentView()
设置收起状态通知的布局。 - 您还可以选择调用
setCustomBigContentView()
为展开式通知设置不同的布局。
准备布局
您需要 small
和 large
布局。在此示例中,small
布局
可能如下所示:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="0dp"
android:layout_weight="1"
android:text="Small notification, showing only a title" />
</LinearLayout>
large
布局可能如下所示:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="300dp"
android:orientation="vertical">
<TextView
android:id="@+id/notification_title"
style="@style/TextAppearance.Compat.Notification.Title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Large notification, showing a title and a body." />
<TextView
android:id="@+id/notification_body"
style="@style/TextAppearance.Compat.Notification.Line2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="This is the body. The height is manually forced to 300dp." />
</LinearLayout>
构建并显示通知
布局准备就绪后,您就可以使用它们,如以下示例所示:
Kotlin
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager // Get the layouts to use in the custom notification. val notificationLayout = RemoteViews(packageName, R.layout.notification_small) val notificationLayoutExpanded = RemoteViews(packageName, R.layout.notification_large) // Apply the layouts to the notification. val customNotification = NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build() notificationManager.notify(666, customNotification)
Java
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); // Get the layouts to use in the custom notification RemoteViews notificationLayout = new RemoteViews(getPackageName(), R.layout.notification_small); RemoteViews notificationLayoutExpanded = new RemoteViews(getPackageName(), R.layout.notification_large); // Apply the layouts to the notification. Notification customNotification = new NotificationCompat.Builder(context, CHANNEL_ID) .setSmallIcon(R.drawable.notification_icon) .setStyle(new NotificationCompat.DecoratedCustomViewStyle()) .setCustomContentView(notificationLayout) .setCustomBigContentView(notificationLayoutExpanded) .build(); notificationManager.notify(666, customNotification);
请注意,通知的背景颜色可能因设备而异
和版本应用支持库样式,例如
TextAppearance_Compat_Notification
适用于文本和
TextAppearance_Compat_Notification_Title
表示自定义布局中的标题,
如以下示例中所示。这些样式会适应颜色变化
最终不会呈现黑底黑字或白底白字的文字。
<TextView android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_weight="1" android:text="@string/notification_title" android:id="@+id/notification_title" style="@style/TextAppearance.Compat.Notification.Title" />
避免在 RemoteViews
对象上设置背景图片,因为其中的文本
都可能变得无法读取
如果您在用户使用应用时触发通知,结果为: 类似于图 1:
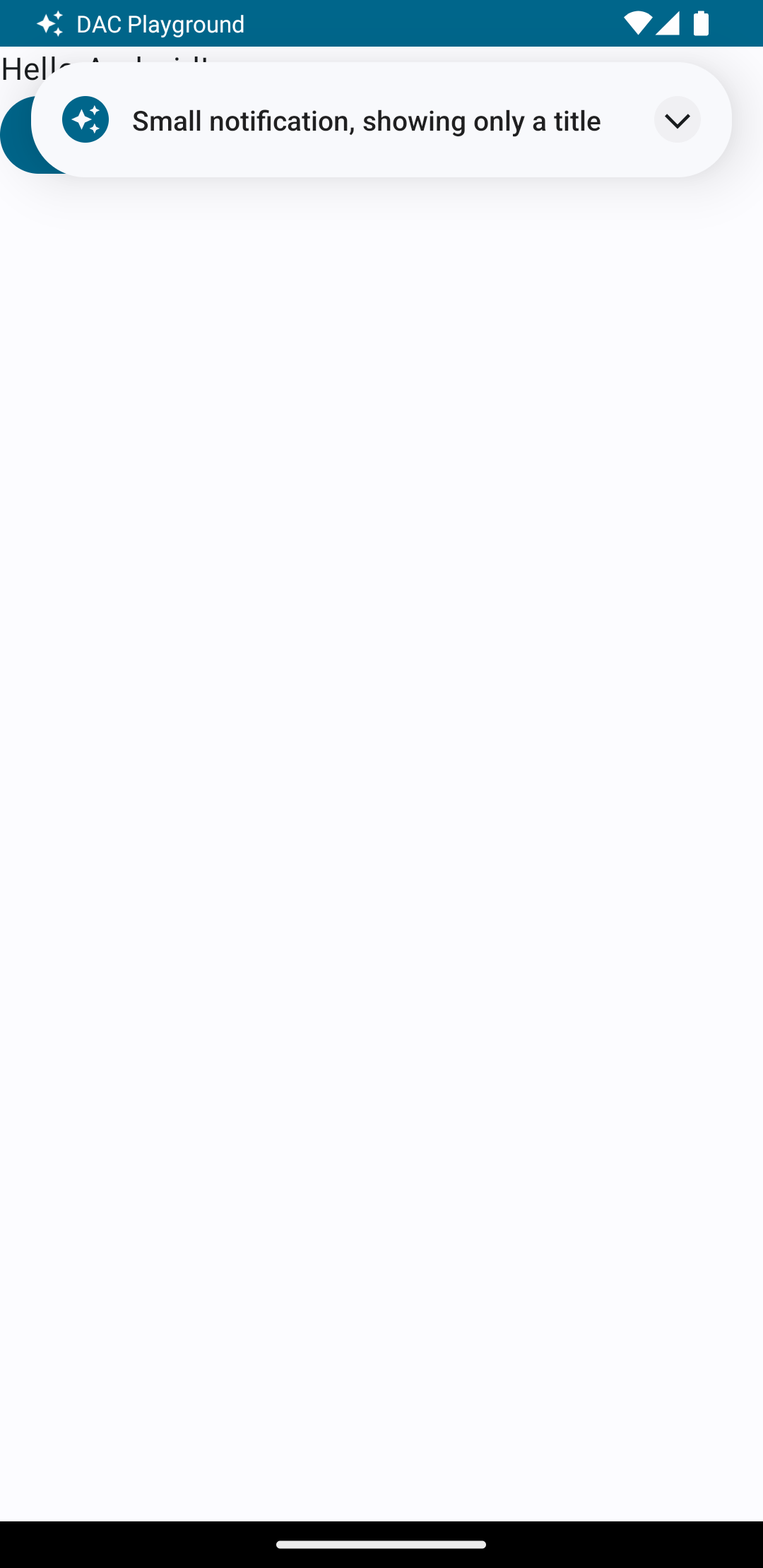
点按展开箭头可展开通知,如图 2 所示:
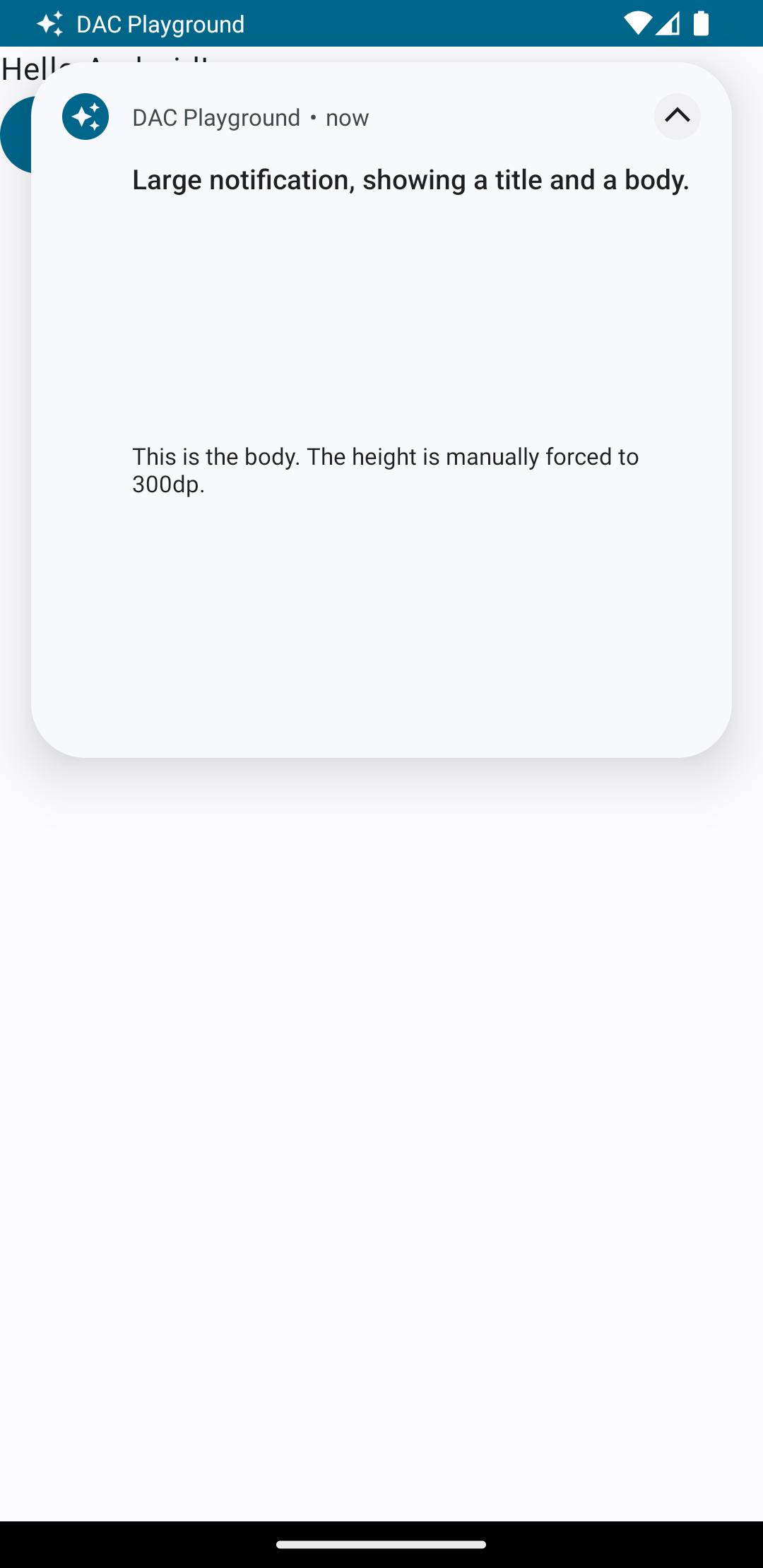
通知超时时间过后,通知将仅在 系统栏,如图 3 所示:
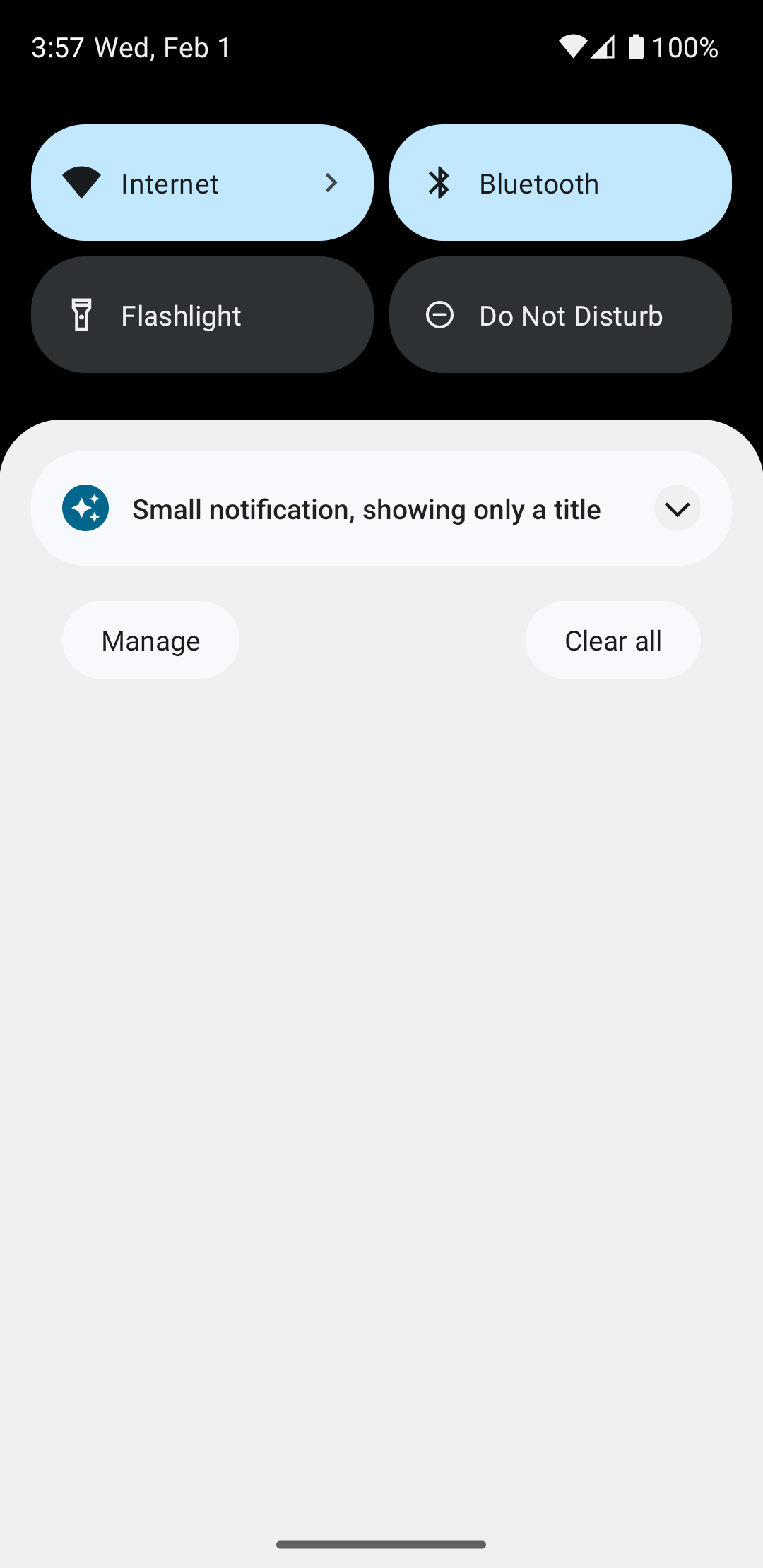
点按展开箭头可展开通知,如图 4 所示:
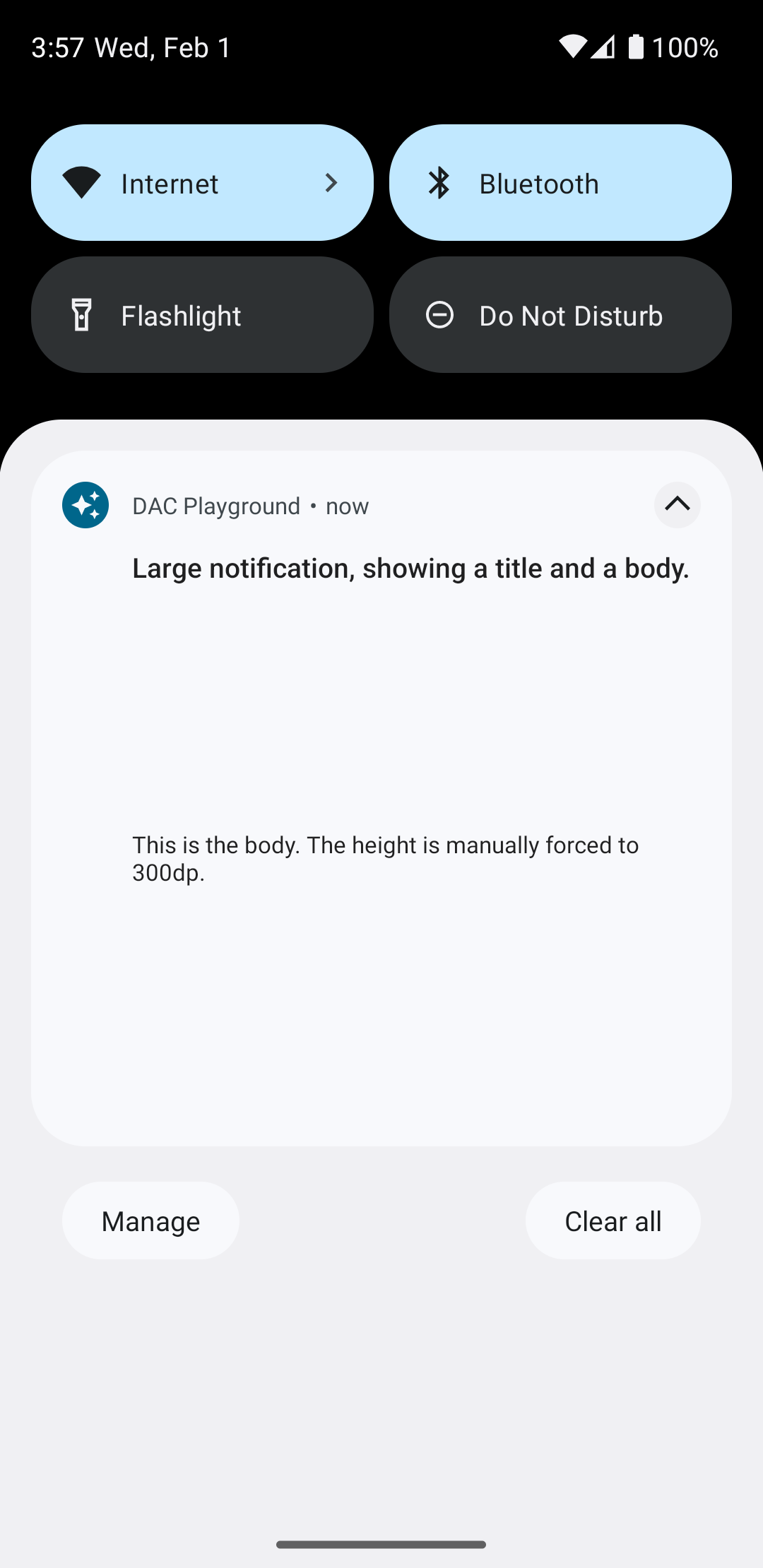
创建完全自定义的通知布局
如果您不想用标准通知装饰通知
图标和标题,请按照上述步骤操作,但不要调用 setStyle()
。