以下部分介绍了如何使用 Glance 创建简单的应用 widget。
在清单中声明 AppWidget
完成设置步骤后,在您的应用中声明 AppWidget
及其元数据。
- 在
AndroidManifest.xml
文件和关联的元数据文件中注册应用 widget 的提供程序:
<receiver android:name=".glance.MyReceiver"
android:exported="true">
<intent-filter>
<action android:name="android.appwidget.action.APPWIDGET_UPDATE" />
</intent-filter>
<meta-data
android:name="android.appwidget.provider"
android:resource="@xml/my_app_widget_info" />
</receiver>
- 从
GlanceAppWidgetReceiver
扩展AppWidget
接收器:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { override val glanceAppWidget: GlanceAppWidget = TODO("Create GlanceAppWidget") }
添加 AppWidgetProviderInfo
元数据
接下来,按照以下步骤添加 AppWidgetProviderInfo
元数据:
按照创建简单的 widget 指南,在
@xml/my_app_widget_info
文件中创建和定义应用 widget 信息。Glance 的唯一区别是没有
initialLayout
XML,但您必须定义一个。您可以使用库中提供的预定义加载布局:
<appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android"
android:initialLayout="@layout/glance_default_loading_layout">
</appwidget-provider>
定义 GlanceAppWidget
创建一个从
GlanceAppWidget
扩展的新类并替换provideGlance
方法。通过此方法,您可以加载渲染 widget 所需的数据:
class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // In this method, load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here Text("Hello World") } } }
- 在
GlanceAppWidgetReceiver
上的glanceAppWidget
中对其进行实例化:
class MyAppWidgetReceiver : GlanceAppWidgetReceiver() { // Let MyAppWidgetReceiver know which GlanceAppWidget to use override val glanceAppWidget: GlanceAppWidget = MyAppWidget() }
您现在已使用 Glance 配置了 AppWidget
。
创建界面
以下代码段演示了如何创建界面:
/* Import Glance Composables In the event there is a name clash with the Compose classes of the same name, you may rename the imports per https://kotlinlang.org/docs/packages.html#imports using the `as` keyword. import androidx.glance.Button import androidx.glance.layout.Column import androidx.glance.layout.Row import androidx.glance.text.Text */ class MyAppWidget : GlanceAppWidget() { override suspend fun provideGlance(context: Context, id: GlanceId) { // Load data needed to render the AppWidget. // Use `withContext` to switch to another thread for long running // operations. provideContent { // create your AppWidget here GlanceTheme { MyContent() } } } @Composable private fun MyContent() { Column( modifier = GlanceModifier.fillMaxSize() .background(GlanceTheme.colors.background), verticalAlignment = Alignment.Top, horizontalAlignment = Alignment.CenterHorizontally ) { Text(text = "Where to?", modifier = GlanceModifier.padding(12.dp)) Row(horizontalAlignment = Alignment.CenterHorizontally) { Button( text = "Home", onClick = actionStartActivity<MainActivity>() ) Button( text = "Work", onClick = actionStartActivity<MainActivity>() ) } } } }
上述代码示例将执行以下操作:
- 在顶层
Column
中,项一个接一个地垂直放置。 Column
会扩展其大小以匹配可用空间(通过GlanceModifier
),并将其内容与顶部对齐 (verticalAlignment
) 并水平居中 (horizontalAlignment
)。Column
的内容使用 lambda 进行定义。顺序很重要。
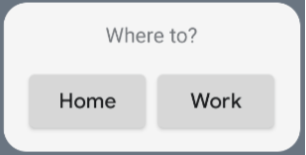
您可以更改对齐值或应用不同的修饰符值(如内边距),以更改组件的位置和尺寸。如需查看每个类的组件、参数和可用修饰符的完整列表,请参阅参考文档。