能够定义要在 OpenGL ES 视图上下文中绘制的形状是 为应用制作高端图形如果不使用 OpenGL ES 绘制, 对 OpenGL ES 期望您如何定义图形对象的一些基本了解。
本课介绍了相对于 Android 设备屏幕、 以及定义三角形和正方形的基本知识。
定义三角形
通过 OpenGL ES,您可以使用三维空间中的坐标定义绘制的对象。因此,
必须先定义其坐标,然后才能绘制三角形。在 OpenGL 中,
这是为坐标定义浮点数的顶点数组。最大
您可以将这些坐标写入 ByteBuffer
,它会传递到
OpenGL ES 图形管道进行处理。
Kotlin
// number of coordinates per vertex in this array const val COORDS_PER_VERTEX = 3 var triangleCoords = floatArrayOf( // in counterclockwise order: 0.0f, 0.622008459f, 0.0f, // top -0.5f, -0.311004243f, 0.0f, // bottom left 0.5f, -0.311004243f, 0.0f // bottom right ) class Triangle { // Set color with red, green, blue and alpha (opacity) values val color = floatArrayOf(0.63671875f, 0.76953125f, 0.22265625f, 1.0f) private var vertexBuffer: FloatBuffer = // (number of coordinate values * 4 bytes per float) ByteBuffer.allocateDirect(triangleCoords.size * 4).run { // use the device hardware's native byte order order(ByteOrder.nativeOrder()) // create a floating point buffer from the ByteBuffer asFloatBuffer().apply { // add the coordinates to the FloatBuffer put(triangleCoords) // set the buffer to read the first coordinate position(0) } } }
Java
public class Triangle { private FloatBuffer vertexBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3; static float triangleCoords[] = { // in counterclockwise order: 0.0f, 0.622008459f, 0.0f, // top -0.5f, -0.311004243f, 0.0f, // bottom left 0.5f, -0.311004243f, 0.0f // bottom right }; // Set color with red, green, blue and alpha (opacity) values float color[] = { 0.63671875f, 0.76953125f, 0.22265625f, 1.0f }; public Triangle() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (number of coordinate values * 4 bytes per float) triangleCoords.length * 4); // use the device hardware's native byte order bb.order(ByteOrder.nativeOrder()); // create a floating point buffer from the ByteBuffer vertexBuffer = bb.asFloatBuffer(); // add the coordinates to the FloatBuffer vertexBuffer.put(triangleCoords); // set the buffer to read the first coordinate vertexBuffer.position(0); } }
默认情况下,OpenGL ES 假定一个坐标系,其中 [0,0,0] (X,Y,Z) 指定
GLSurfaceView
帧,
[1,1,0] 表示帧的右上角,
[-1,-1,0] 是框架的左下角。有关此坐标系的图示,请参阅
OpenGL ES 开发者
指南。
请注意,此形状的坐标是按照逆时针顺序定义的。绘图 顺序很重要,因为它定义了形状的哪一侧是正面,通常 以及背面(您可以选择不通过 OpenGL ES 剔除功能进行绘制) 人脸识别功能。有关面和剔除的详情,请参阅 OpenGL ES 开发者指南。
定义方形
在 OpenGL 中定义三角形非常简单,但如果您想多获取一点,该怎么办? 复杂?假设定义方形呢?有多种方法可以实现这一目的,但绘制此类 即使用两个一起绘制的三角形:
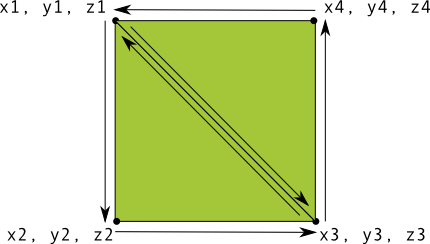
图 1. 使用两个三角形绘制一个方形。
同样,您应当按逆时针顺序定义两个三角形的顶点,
代表该形状,并将值放入 ByteBuffer
中。为了避免
定义每个三角形共享的两个坐标两次,可以使用绘图列表告诉
OpenGL ES 图形管道如何绘制这些顶点。下面是此形状的代码:
Kotlin
// number of coordinates per vertex in this array const val COORDS_PER_VERTEX = 3 var squareCoords = floatArrayOf( -0.5f, 0.5f, 0.0f, // top left -0.5f, -0.5f, 0.0f, // bottom left 0.5f, -0.5f, 0.0f, // bottom right 0.5f, 0.5f, 0.0f // top right ) class Square2 { private val drawOrder = shortArrayOf(0, 1, 2, 0, 2, 3) // order to draw vertices // initialize vertex byte buffer for shape coordinates private val vertexBuffer: FloatBuffer = // (# of coordinate values * 4 bytes per float) ByteBuffer.allocateDirect(squareCoords.size * 4).run { order(ByteOrder.nativeOrder()) asFloatBuffer().apply { put(squareCoords) position(0) } } // initialize byte buffer for the draw list private val drawListBuffer: ShortBuffer = // (# of coordinate values * 2 bytes per short) ByteBuffer.allocateDirect(drawOrder.size * 2).run { order(ByteOrder.nativeOrder()) asShortBuffer().apply { put(drawOrder) position(0) } } }
Java
public class Square { private FloatBuffer vertexBuffer; private ShortBuffer drawListBuffer; // number of coordinates per vertex in this array static final int COORDS_PER_VERTEX = 3; static float squareCoords[] = { -0.5f, 0.5f, 0.0f, // top left -0.5f, -0.5f, 0.0f, // bottom left 0.5f, -0.5f, 0.0f, // bottom right 0.5f, 0.5f, 0.0f }; // top right private short drawOrder[] = { 0, 1, 2, 0, 2, 3 }; // order to draw vertices public Square() { // initialize vertex byte buffer for shape coordinates ByteBuffer bb = ByteBuffer.allocateDirect( // (# of coordinate values * 4 bytes per float) squareCoords.length * 4); bb.order(ByteOrder.nativeOrder()); vertexBuffer = bb.asFloatBuffer(); vertexBuffer.put(squareCoords); vertexBuffer.position(0); // initialize byte buffer for the draw list ByteBuffer dlb = ByteBuffer.allocateDirect( // (# of coordinate values * 2 bytes per short) drawOrder.length * 2); dlb.order(ByteOrder.nativeOrder()); drawListBuffer = dlb.asShortBuffer(); drawListBuffer.put(drawOrder); drawListBuffer.position(0); } }
通过此示例,您可以简单了解如何使用 OpenGL 绘制比较复杂的形状。在 一般可以使用三角形的集合来绘制对象。在下一课中,您将学习如何绘制 这些形状在屏幕上