有时,您的布局需要很少使用的复杂视图。无论 它们是商品详情、进度指示器或撤消消息,您可以减少 并加快渲染速度,因为只有在 所需的资源。
如果您的应用具有复杂的视图,您可以延迟加载
来预测未来的需求,
ViewStub
:
复杂且很少使用的视图。
定义 ViewStub
ViewStub
是一种没有任何维度的轻量级视图,
绘制任何内容或参与布局。因此,它只需要很少的资源
膨胀并留在视图层次结构中每个 ViewStub
均包含
android:layout
属性,用于指定要膨胀的布局。
假设您有一个布局想要在稍后的用户体验历程中加载 app:
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:src="@drawable/logo" android:layout_width="match_parent" android:layout_height="match_parent"/> </FrameLayout>
您可以使用以下 ViewStub
推迟加载。为了让
它显示或加载任何内容,您必须使其显示引用的布局:
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/root" android:layout_width="match_parent" android:layout_height="match_parent"> <ViewStub android:id="@+id/stub_import" android:inflatedId="@+id/panel_import" android:layout="@layout/heavy_layout_we_want_to_postpone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="bottom" /> </FrameLayout>
加载 ViewStub 布局
上一部分中的代码段会产生类似于图 1:
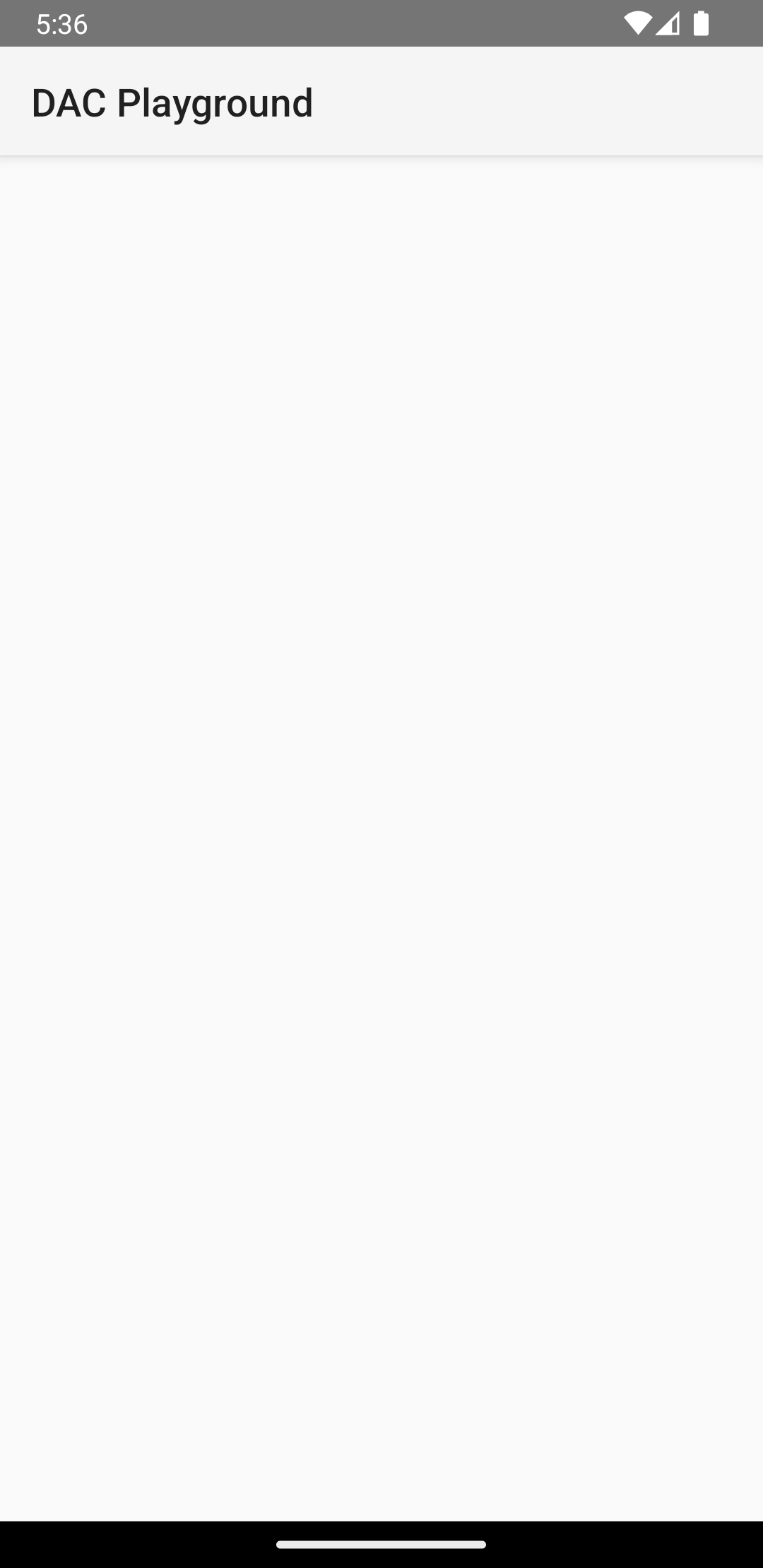
ViewStub
为
隐藏了沉重布局当您需要加载 ViewStub
指定的布局时,
通过调用以下代码将其设为可见
setVisibility(View.VISIBLE)
或致电
inflate()
。
以下代码段模拟了延迟加载。屏幕加载方式为
Activity
和onCreate()
的正常价格,
heavy_layout_we_want_to_postpone
布局:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_old_xml) Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000) }
Java
@Override void onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_old_xml); Handler(Looper.getMainLooper()) .postDelayed({ findViewById<View>(R.id.stub_import).visibility = View.VISIBLE // Or val importPanel: View = findViewById<ViewStub>(R.id.stub_import).inflate() }, 2000); }
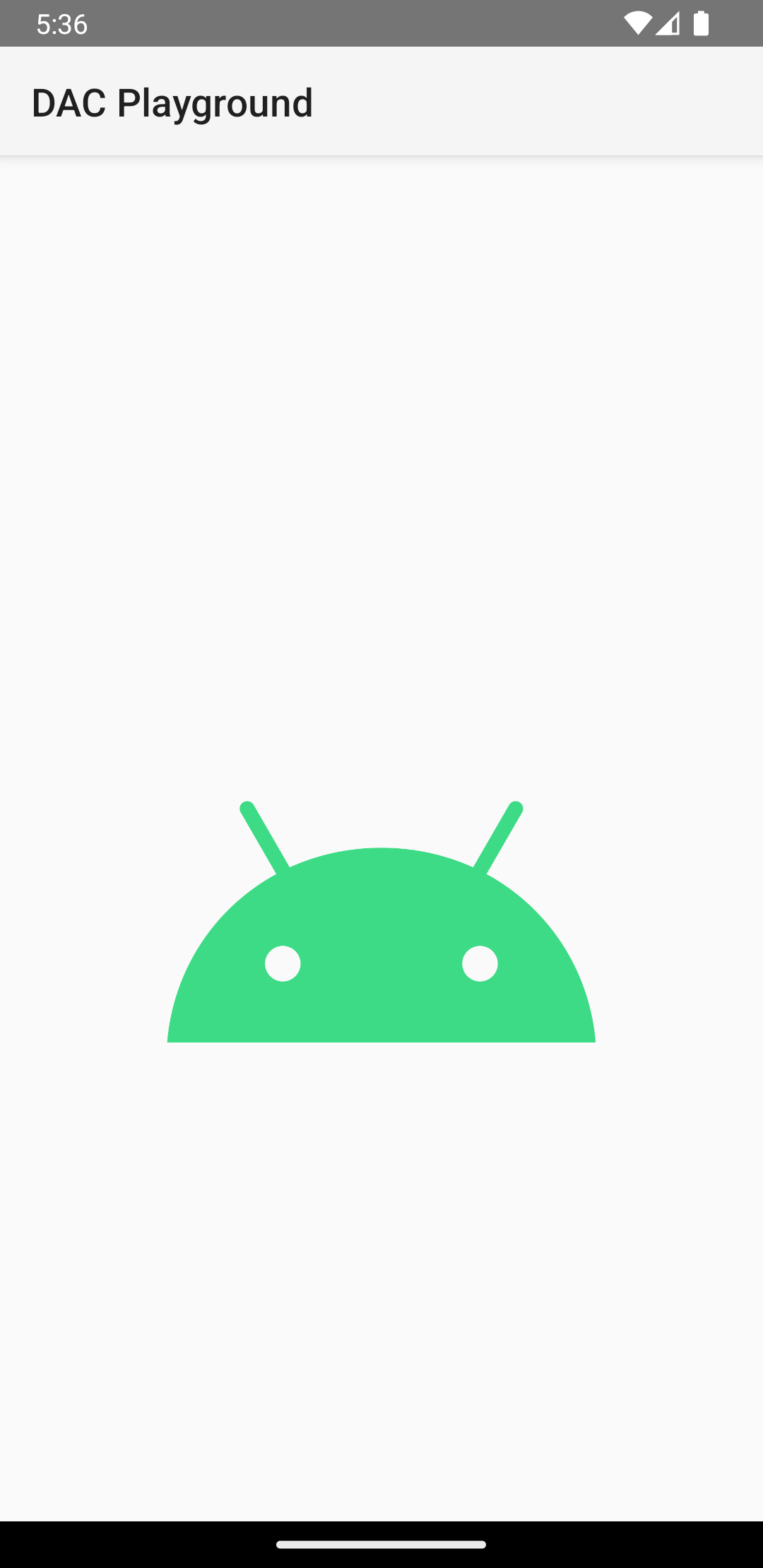
ViewStub
元素一旦可见或膨胀,便不再是它的一部分
视图层次结构的其中一个部分它会被替换为经过膨胀的布局、
该布局的根视图由 android:inflatedId
指定
ViewStub
属性的值。指定的 ID android:id
对于 ViewStub
仅在 ViewStub
可见或膨胀。
如需详细了解此主题,请参阅此博文 优化 使用桩。