The navigation bar allows users to switch between destinations in an app. You should use navigation bars for:
- Three to five destinations of equal importance
- Compact window sizes
- Consistent destinations across app screens
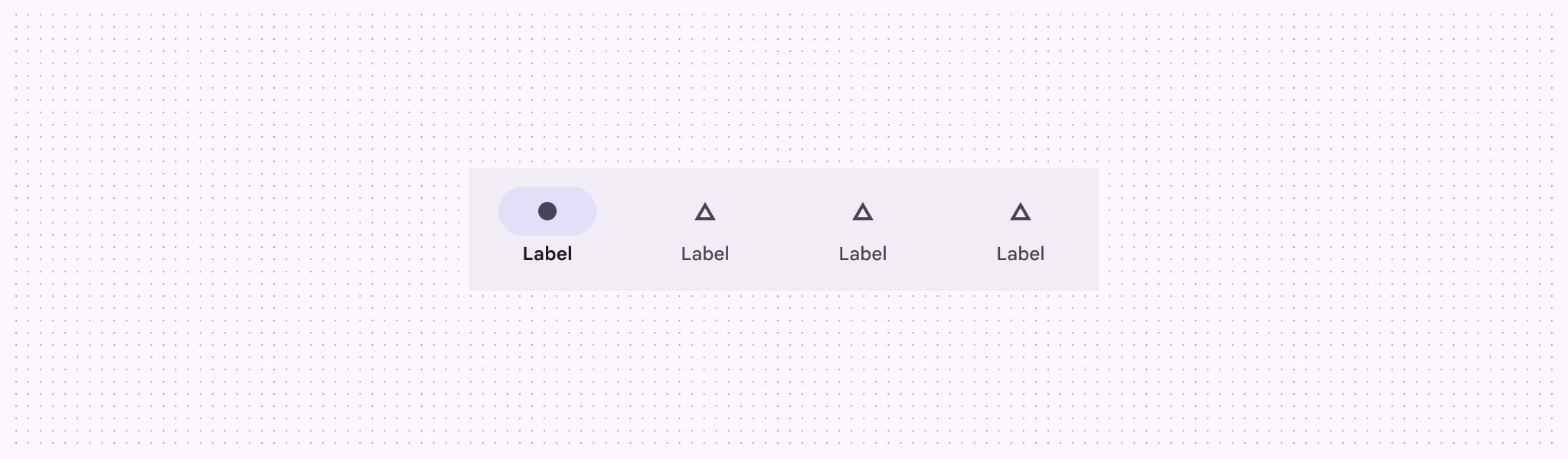
This page shows you how to display a navigation bar in your app with related screens and basic navigation.
API surface
Use the NavigationBar
and NavigationBarItem
composables to
implement destination switching logic. Each NavigationBarItem
represents a
singular destination.
NavigationBarItem
includes the following key parameters:
selected
: Determines whether the current item is visually highlighted.onClick()
: A required lambda function that defines the action to be performed when the user clicks on the item. This is where you typically handle navigation events, update the selected item state, or load corresponding content.label
: Displays text within the item. Optional.icon
: Displays an icon within the item. Optional.
Example: Bottom navigation bar
The following snippet implements a bottom navigation bar with items so users can navigate between different screens in an app:
@Composable fun NavigationBarExample(modifier: Modifier = Modifier) { val navController = rememberNavController() val startDestination = Destination.SONGS var selectedDestination by rememberSaveable { mutableIntStateOf(startDestination.ordinal) } Scaffold( modifier = modifier, bottomBar = { NavigationBar(windowInsets = NavigationBarDefaults.windowInsets) { Destination.entries.forEachIndexed { index, destination -> NavigationBarItem( selected = selectedDestination == index, onClick = { navController.navigate(route = destination.route) selectedDestination = index }, icon = { Icon( destination.icon, contentDescription = destination.contentDescription ) }, label = { Text(destination.label) } ) } } } ) { contentPadding -> AppNavHost(navController, startDestination, modifier = Modifier.padding(contentPadding)) } }
Key points
NavigationBar
displays a collection of items, with each item corresponding to aDestination
.val navController = rememberNavController()
creates and remembers an instance ofNavHostController
, which manages the navigation within aNavHost
.var selectedDestination by rememberSaveable { mutableIntStateOf(startDestination.ordinal)
} manages the state of the currently selected item.var selectedDestination by rememberSaveable { mutableIntStateOf(startDestination.ordinal) }
manages the state of the currently selected item.startDestination.ordinal
gets the numerical index (position) of theDestination.SONGS
enum entry.
- When an item is clicked,
navController.navigate(route = destination.route)
is called to navigate to the corresponding screen. - The
onClick
lambda of theNavigationBarItem
updates theselectedDestination
state to visually highlight the clicked item. - It calls the
AppNavHost
composable, passing thenavController
andstartDestination
, to display the actual content of the selected screen.
Result
The following image shows the navigation bar resulting from the previous snippet:
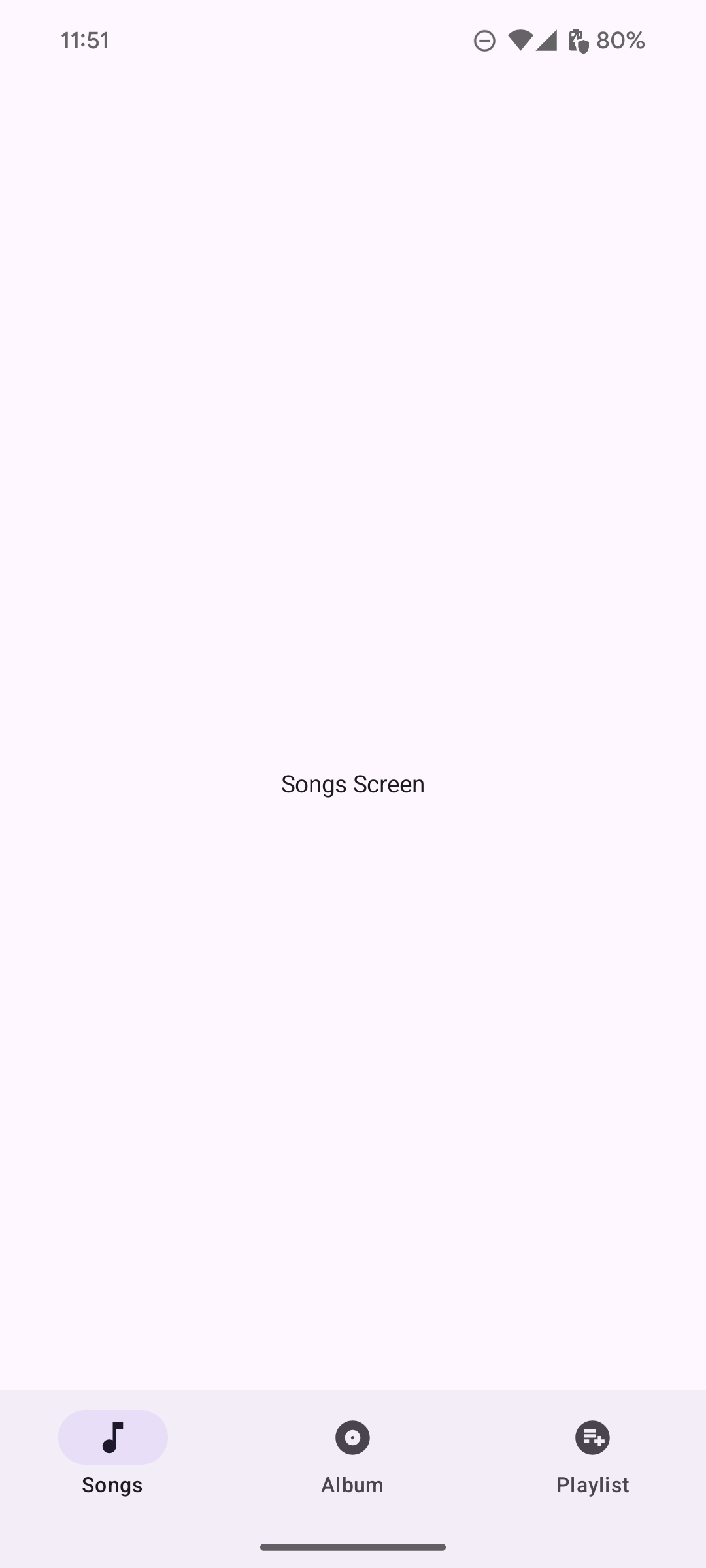