使用提示为按钮或其他界面元素添加上下文。提示有两种类型:
- 普通提示:描述图标按钮的元素或操作。
- 丰富的提示:提供更多详细信息,例如描述地图项的价值。还可以包含可选的标题、链接和按钮。
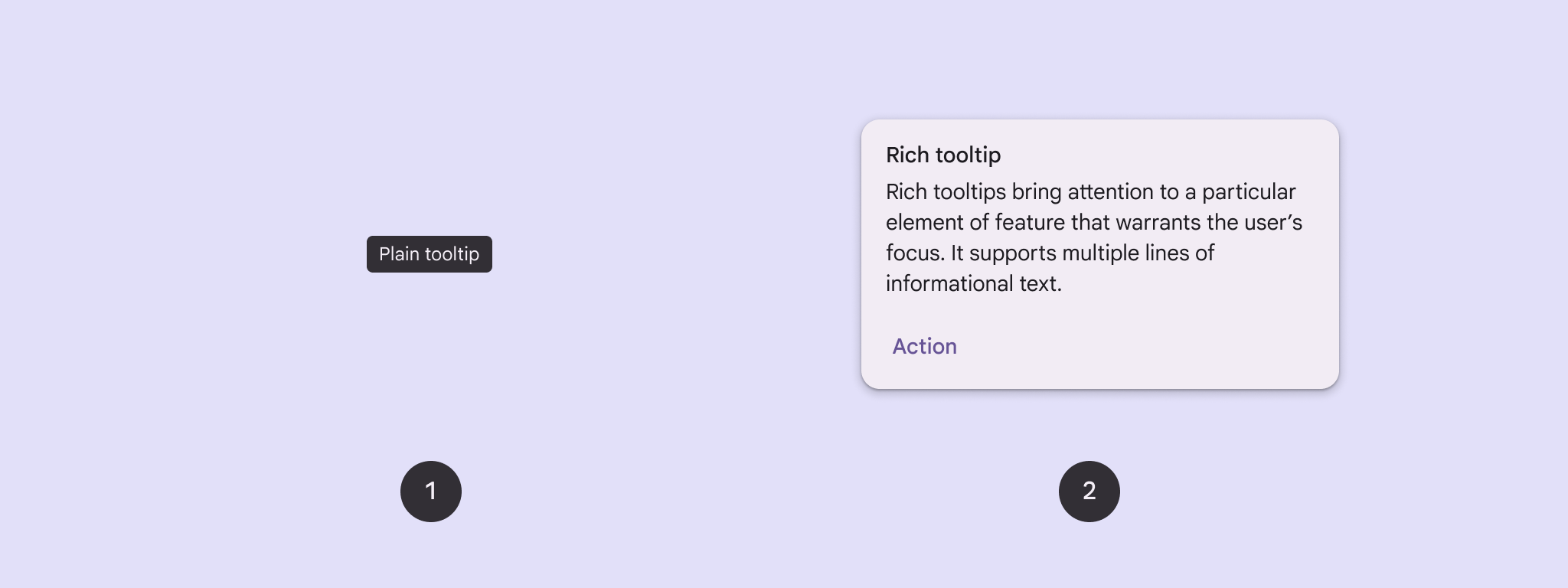
API Surface
您可以使用 TooltipBox
可组合项在应用中实现提示。您可以使用以下主要参数控制 TooltipBox
的外观:
positionProvider
:相对于锚点内容放置提示。您通常使用TooltipDefaults
中的默认位置提供程序,如果需要自定义定位逻辑,也可以提供自己的位置提供程序。tooltip
:包含提示内容的可组合项。您通常使用PlainTooltip
或RichTooltip
可组合项。- 使用
PlainTooltip
描述图标按钮的元素或操作。 - 使用
RichTooltip
提供更多详细信息,例如描述某个功能的价值。富文本提示可以包含可选的标题、链接和按钮。
- 使用
state
:包含此提示的界面逻辑和元素状态的状态容器。content
:悬停提示锚定的可组合项内容。
显示普通提示
使用普通的提示来简要说明界面元素。以下代码段会在标签为“添加到收藏夹”的图标按钮上方显示一个普通提示:
@Composable fun PlainTooltipExample( modifier: Modifier = Modifier, plainTooltipText: String = "Add to favorites" ) { TooltipBox( modifier = modifier, positionProvider = TooltipDefaults.rememberPlainTooltipPositionProvider(), tooltip = { PlainTooltip { Text(plainTooltipText) } }, state = rememberTooltipState() ) { IconButton(onClick = { /* Do something... */ }) { Icon( imageVector = Icons.Filled.Favorite, contentDescription = "Add to favorites" ) } } }
代码要点
TooltipBox
会生成一条内容为“添加到收藏夹”的提示。TooltipDefaults.rememberPlainTooltipPositionProvider()
为普通提示提供了默认定位。tooltip
是一个 lambda 函数,用于使用PlainTooltip
可组合项定义提示内容。Text(plainTooltipText)
会在提示中显示文本。tooltipState
用于控制提示的状态。
IconButton
用于创建带有图标的可点击按钮。Icon(...)
会在按钮中显示一个心形图标。- 当用户与
IconButton
互动时,TooltipBox
会显示包含“添加到收藏夹”文本的提示。用户可以通过以下方式触发提示(具体取决于设备): - 将光标悬停在图标上
- 在移动设备上长按该图标
结果
此示例会在图标上方生成普通提示:
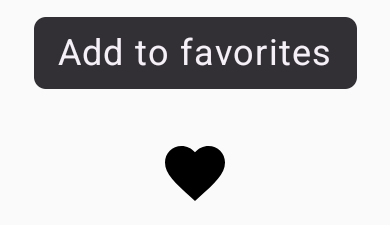
显示富文本提示
使用丰富的提示提供有关界面元素的其他背景信息。以下示例会创建一个多行富文本提示,其标题锚定到 Icon
:
@Composable fun RichTooltipExample( modifier: Modifier = Modifier, richTooltipSubheadText: String = "Rich Tooltip", richTooltipText: String = "Rich tooltips support multiple lines of informational text." ) { TooltipBox( modifier = modifier, positionProvider = TooltipDefaults.rememberRichTooltipPositionProvider(), tooltip = { RichTooltip( title = { Text(richTooltipSubheadText) } ) { Text(richTooltipText) } }, state = rememberTooltipState() ) { IconButton(onClick = { /* Icon button's click event */ }) { Icon( imageVector = Icons.Filled.Info, contentDescription = "Show more information" ) } } }
代码要点
TooltipBox
会处理用户互动的事件监听器,并相应地更新TooltipState
。当TooltipState
指示应显示提示时,系统会执行提示 lambda,并通过TooltipBox
显示RichTooltip
。TooltipBox
充当内容和提示的锚点和容器。- 在本例中,内容是
IconButton
组件,它提供可点按的操作行为。当您在TooltipBox
内容中的任意位置长按(在触摸设备上)或悬停(如使用鼠标指针)时,系统会显示提示以显示更多信息。
- 在本例中,内容是
RichTooltip
可组合项用于定义提示内容,包括标题和正文。TooltipDefaults.rememberRichTooltipPositionProvider()
可为富文本提示提供定位信息。
结果
以下示例会生成一个富文本提示,其中标题附加在信息图标上:
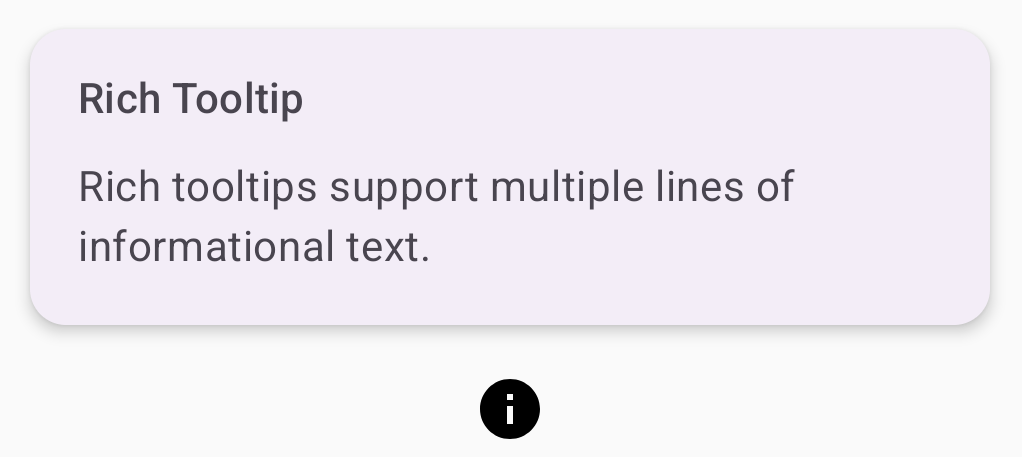
自定义富文本提示
以下代码段会显示一个富有内容的提示,其中包含标题、自定义操作,以及在相机图标按钮上显示的自定义箭头:
@Composable fun AdvancedRichTooltipExample( modifier: Modifier = Modifier, richTooltipSubheadText: String = "Custom Rich Tooltip", richTooltipText: String = "Rich tooltips support multiple lines of informational text.", richTooltipActionText: String = "Dismiss" ) { val tooltipState = rememberTooltipState() val coroutineScope = rememberCoroutineScope() TooltipBox( modifier = modifier, positionProvider = TooltipDefaults.rememberRichTooltipPositionProvider(), tooltip = { RichTooltip( title = { Text(richTooltipSubheadText) }, action = { Row { TextButton(onClick = { coroutineScope.launch { tooltipState.dismiss() } }) { Text(richTooltipActionText) } } }, caretSize = DpSize(32.dp, 16.dp) ) { Text(richTooltipText) } }, state = tooltipState ) { IconButton(onClick = { coroutineScope.launch { tooltipState.show() } }) { Icon( imageVector = Icons.Filled.Camera, contentDescription = "Open camera" ) } } }
代码要点
RichToolTip
会显示包含标题和关闭操作的提示。- 启用后(通过长按或将鼠标指针悬停在
ToolTipBox
内容上),工具提示会显示大约一秒钟。您可以点按屏幕上的其他位置或使用关闭操作按钮关闭此提示。 - 执行关闭操作时,系统会启动协程来调用
tooltipState.dismiss
。这可验证在显示提示时操作执行是否不会被阻止。 onClick = coroutineScope.launch { tooltipState.show() } }
会启动协程,以使用tooltipState.show
手动显示提示。action
参数允许向提示中添加互动元素,例如按钮。caretSize
参数用于修改提示箭头的大小。
结果
此示例会生成以下内容:
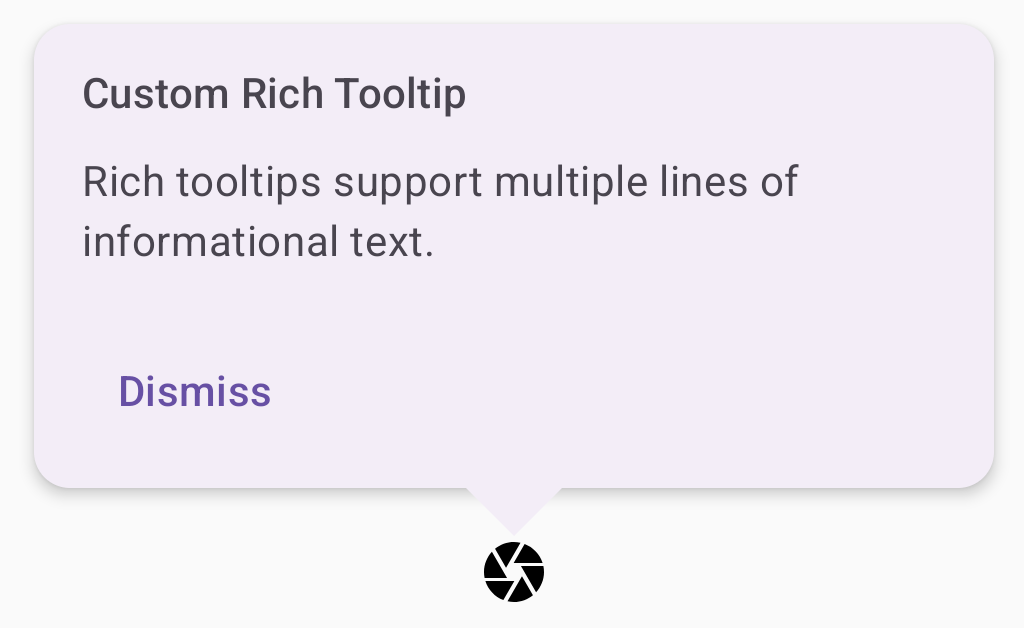
其他资源
- Material Design:提示